TL;DR
article explains how to avoid slowing down your web app by canceling or aborting previous AJAX requests. It provides a code snippet that assigns the current AJAX request object to a variable and checks if it’s complete before initiating a new request, thus aborting the previous one. The article also includes a solution for ignoring the “abort” error that occurs when a previous request is canceled.
jQuery AJAX is the most common way to deal with AJAX requests these days. You can send as many Ajax requests as you like but there are many cases in which sending too many or frequent Ajax requests is not suitable and best avoided.
For example, let’s say you have developed a certain search functionality that fetches result data using AJAX. The search query string is the value of the input field bound with the Ajax function and is triggered on the keyup event on the input field. In this case, if the user has to enter a query string of let’s say 8 characters, this will mean that there’ll be at least 8 keyup events triggered, which further means that there’ll be at least 8 Ajax calls. This will definitely lead to a slowing of the whole app and is unreasonable as well.
The solution here is to only focus on the query string after the user has finished typing i.e. the last keyup event. So, for this, you have to cancel or abort all the previous Ajax requests.
Let’s say that the input field is as follows:
[PHP]
[/php]
Now, we have to write the code for the Ajax function which will be triggered on the key up event and will also abort the previous Ajax calls if any. So, our code for such a function will be as:
In the above code what’s happening is that we have assigned our $.ajax request to a variable ‘ajaxReq‘. This variable will hold the current Ajax request object and if another Ajax request is called before the previous one is completed it aborts the previous one. It does this by checking the condition set in the beforeSend function which fires before a new Ajax request is initiated.
The ajaxReq != ‘ToCancelPrevReq’ checks if the ajaxReq variable holds a previous ajax request object and the ajaxReq.readyState < 4 condition checks if that is complete or not. The readyState property can have 5 values depending on the status of the Ajax XMLHttpRequest, these are:
1: server connection established
2: request received
3: processing request
4: request finished and response is ready
You can learn more about XMLHttpRequest at:
https://www.w3schools.com/xml/ajax_xmlhttprequest_create.asp
The condition:
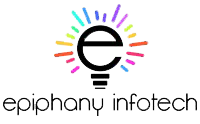
We are a Website Development, WebApp Development, and Digital Marketing Company, providing services to enterprises of all shapes and sizes, across the world. In our blog, we talk about the latest in Tech, Technical Tutorials, and our general opinions, among other things 🙂 Please feel free to reach us through the comment box or via the contact us page if you’d like to know more about our services.